所謂的Git Hooks指的是以腳本等方式設定執行動作,當git指令執行時,會自動觸發這些腳本的機制,時機可能是在git指令之前或是之後。根據git指令又可以分為server side和client slide兩大類:commit和merge觸發的是client side,server side則是在網路上收到推上去的commit(pushed commit)。
hooks 存放在目錄 /.git/hooks/ 裡,執行 git init 初始化時,git自動在目錄裡產生一些shell script範本,你也可以用Ruby或python等實作。範例可以直接使用,但要記得把副檔名的 .sample 拿掉。
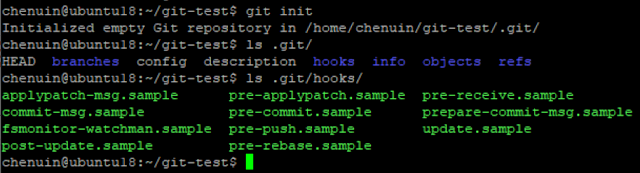
如果需要自己新建hook,請確認一下檔案的執行權限已經開啟。
chmod +x [filePath]
常見的Hooks
Client Side
以下為4個與commit相關的hook:- 在commit之前會執行 pre-commit,可以運用在執行功能驗證、單元測試或是coding style的檢查,若有任何錯誤發生時,這個commit會被捨棄不執行。也可以下指令 git commit --no-verify 略過hook的執行。
- prepare-commit-msg,這個hook對於一般commit比較不實用,多在commit訊息模板、merge commit、squash commits、amended commits等自動產生commit訊息的類型。
- commit-msg需要commit訊息的模板檔案路徑作為參數,如果錯誤發生,git會停止進行這個commit,來確保所有的commit訊息都符合需要的格式。
- commit結束後,會執行 post-commit ,不需要任何參數,你可以用 git log -1 HEAD 來確認剛剛commit的內容,或者通知其他類似的訊息。
Server Side
- server端收到push時,首先執行 pre-receive ,如果錯誤發生,所有的commit將不會被受理。可以用來檢查有沒有任何non-fast-forwards。
- update 和 pre-receive 有點相似。
- post-receive 會在整個push過程結束後執行,可以用來通知專案成員或是其他服務的更新等。這個hook不會影響push過程,但在hook執行完之前,用戶端和伺服器端會持續連線,因此要避免在腳本中執行時間較長的工作。
參考資料:
https://git-scm.com/book/zh-tw/v2/Customizing-Git-Git-Hooks